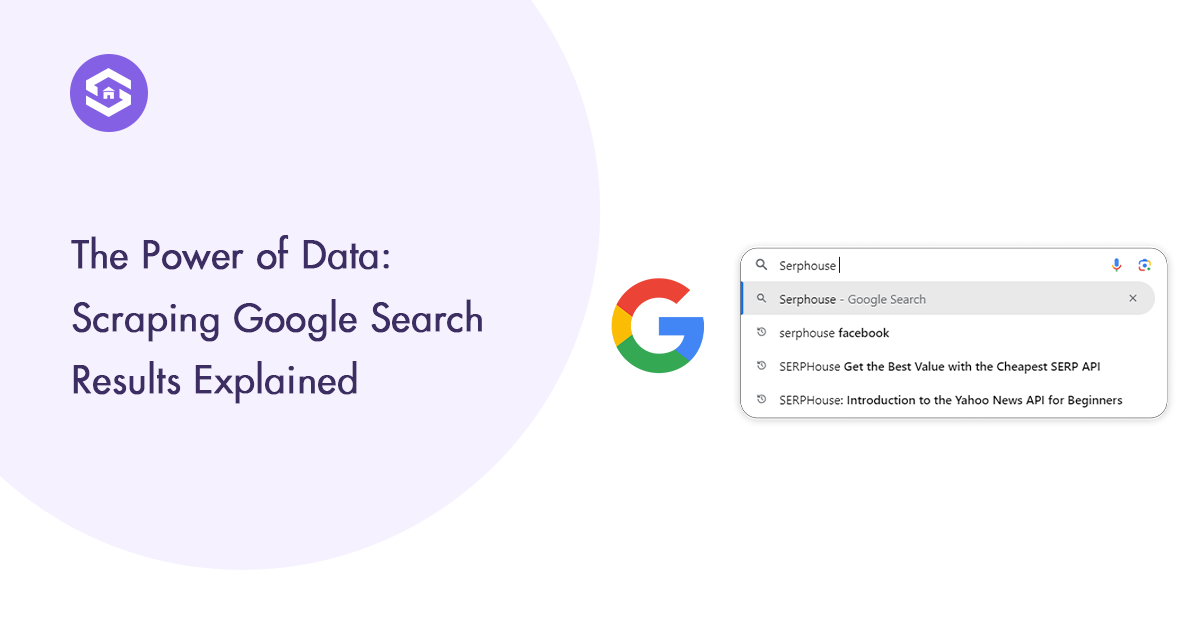
Web scraping involves extracting data from websites. This automated process can capture large amounts of data quickly, making it an invaluable tool for tasks like Scraping Google Search Results, keyword rank tracking, competitor analysis based on SERP data, and market research.
Why Scraping Google Search Results?
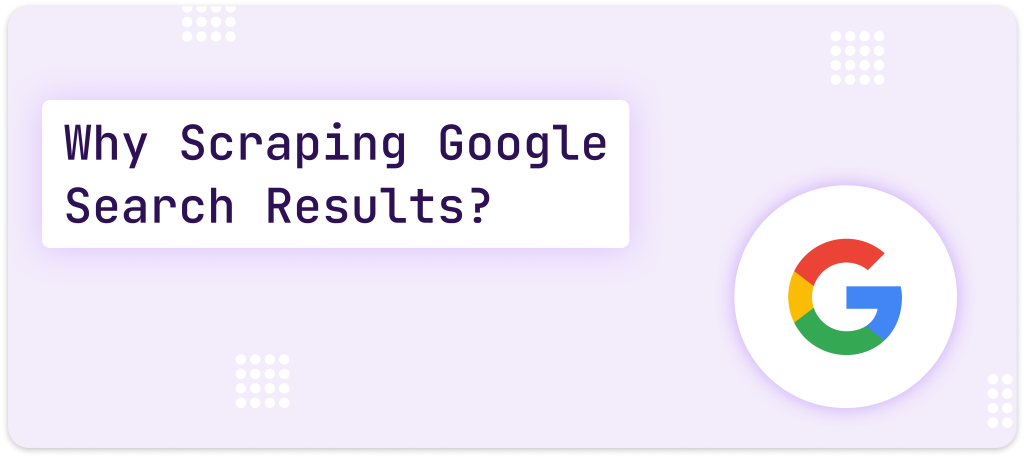
Scraping Google search results provides several benefits:
- Keyword Rank Tracking: Monitor your website’s search engine ranking for targeted keywords.
- Competitor Analysis: Gather data on competitors’ performance and strategies.
- Market Research: Identify trends and insights within your industry.
Legal and Ethical Considerations
Before proceeding with web scraping, it’s essential to understand the legal and ethical implications. Google’s terms of service prohibit automated scraping. However, there are ethical approaches, such as using official APIs or ensuring compliance with robots.txt files.
Getting Started with Web Scraping
Several tools and libraries can facilitate web scraping. One prominent option is SERPHouse, a robust API designed for extracting search engine results.
Using SERPHouse for Web Scraping
SERPHouse is a powerful API that simplifies extracting Google search results. It provides accurate and reliable data, making it a valuable asset for SEO professionals and businesses.
Key Features of SERPHouse:
- Real-time data extraction
- Support for multiple search engines
- Ability to track keyword position
- Easy integration with various programming languages
Getting Started with SERPHouse:
- Sign Up: Create an account on the SERPHouse website.
- API Key: Obtain your API key from the dashboard.
- Integration: Use the API key to integrate SERPHouse with your scraping script.
Sample Code Using SERPHouse API:
import requests
api_key = 'your_serphouse_api_key'
query = 'web scraping'
url = f"https://api.serphouse.com/?api_key={api_key}&q={query}"
response = requests.get(url)
data = response.json()
for result in data['results']:
title = result['title']
link = result['link']
snippet = result['snippet']
print(f"Title: {title}\nLink: {link}\nSnippet: {snippet}\n")
Other Popular Tools and Libraries
While SERPHouse is a specialized tool for SERP data, other general-purpose web scraping tools and libraries can also be used:
- Beautiful Soup: Python library designed for parsing HTML and XML documents.
- Scrapy: An open-source web crawling framework for Python.
- Selenium: A tool for automating web browsers to scrape dynamic content.
Step-by-Step Guide to Web Scraping Google Search Results
Setting Up Your Environment
Before you start scraping, set up your development environment. Here’s a quick guide for Python:
pip install beautifulsoup4
pip install requests
pip install selenium
Fetching the Web Page
Use the requests library to fetch the Google search results page:
import requests
query = 'web scraping'
url = f"https://www.google.com/search?q={query}"
response = requests.get(url)
html_content = response.text
Parsing the HTML
Use Beautiful Soup to parse the HTML content and extract the desired data:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
search_results = soup.find_all('div', class_='g')
for result in search_results:
title = result.find('h3').text
link = result.find('a')['href']
snippet = result.find('span', class_='aCOpRe').text
print(f"Title: {title}\nLink: {link}\nSnippet: {snippet}\n")
Handling Dynamic Content
If you need to scrape dynamic content, Selenium can be useful:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get(url)
html_content = driver.page_source
driver.quit()
soup = BeautifulSoup(html_content, 'html.parser')
# Continue parsing as before
Advanced Techniques
Using Proxies
To avoid being blocked by Google, use proxies to distribute your requests:
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
response = requests.get(url, proxies=proxies)
Rate Limiting
Implement rate limiting to prevent your IP from being blocked:
import time
for query in queries:
url = f"https://www.google.com/search?q={query}"
response = requests.get(url)
time.sleep(2) # Wait for 2 seconds
Data Storage
Once you have scraped the data, store it for further analysis. Options include CSV files, databases, or other storage solutions.
Storing in a CSV File
import csv
with open('search_results.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(["Title", "Link", "Snippet"])
for result in search_results:
writer.writerow([result['title'], result['link'], result['snippet']])
Storing in a Database
For more complex projects, storing data in a database might be more suitable. Using a database allows for efficient querying and management of large datasets.
import sqlite3
# Connect to SQLite database
conn = sqlite3.connect('search_results.db')
c = conn.cursor()
# Create table
c.execute('''CREATE TABLE results
(title TEXT, link TEXT, snippet TEXT)''')
# Insert data
for result in search_results:
c.execute("INSERT INTO results (title, link, snippet) VALUES (?, ?, ?)",
(result['title'], result['link'], result['snippet']))
# Save (commit) the changes and close the connection
conn.commit()
conn.close()
Use Cases for Web Scraping Google Search Results
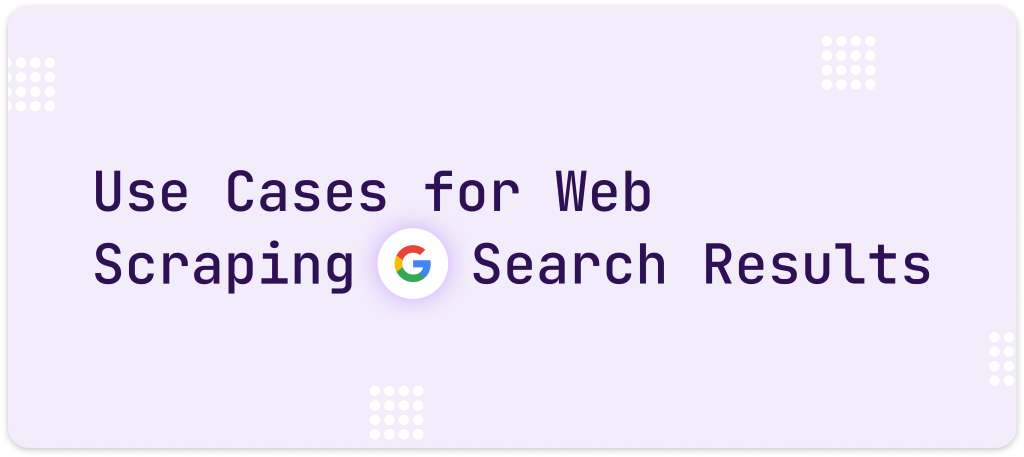
Keyword Rank Tracking
Monitoring your website’s position for various keywords over time is crucial for assessing the effectiveness of your SEO efforts and making necessary adjustments. By scraping Google search results, you can track keyword rankings and identify trends.
Competitor Analysis
Scraping SERP data provides insights into your competitors’ strategies, such as the keywords they are targeting and the type of content they produce. This information can inform your own marketing and content strategies, helping you stay competitive.
Market Research
Gathering data on market trends, customer preferences, and industry insights through web scraping can help you make informed business decisions and stay ahead of the competition. Analyzing this data enables you to identify opportunities and optimize your strategies.
Challenges and Solutions
Captchas
Google uses Captchas to prevent automated access. One way to handle this is to use a service like Captcha to solve captchas programmatically. Additionally, rotating user agents and implementing smart scraping strategies can reduce the frequency of Captchas.
IP Blocking
Frequent requests from a single IP address can lead to blocking. Using proxies and implementing rate limiting can mitigate this issue. Rotating proxies and distributing requests over time can help maintain access to the desired data.
Changing HTML Structure
Web pages frequently change their structure, which can break your scraper. Regularly updating your scraping scripts to handle these changes is essential. Using more robust parsers and adaptable scraping techniques can help maintain the functionality of your scraper.
Conclusion
Web scraping Google search results is a powerful technique for gaining insights into keyword rank tracking, competitor analysis, and market research. By using the right tools and following best practices, you can extract valuable data to inform your business strategies. Always consider the legal and ethical implications of web scraping, and use these techniques responsibly.
In this guide, we’ve covered the basics of web scraping, provided a step-by-step tutorial, and discussed advanced techniques and use cases. Whether you’re a beginner or an experienced data analyst, this comprehensive guide will help you harness the power of web scraping to achieve your goals.
By integrating tools like SERPHouse and leveraging libraries such as Beautiful Soup, Scrapy, and Selenium, you can efficiently scrape and analyze Google search results. Remember to handle challenges like Captchas, IP blocking, and changing HTML structures proactively to ensure the effectiveness of your web scraping efforts.